Maximize Function Reusability: Parameterization For Flexible Functions
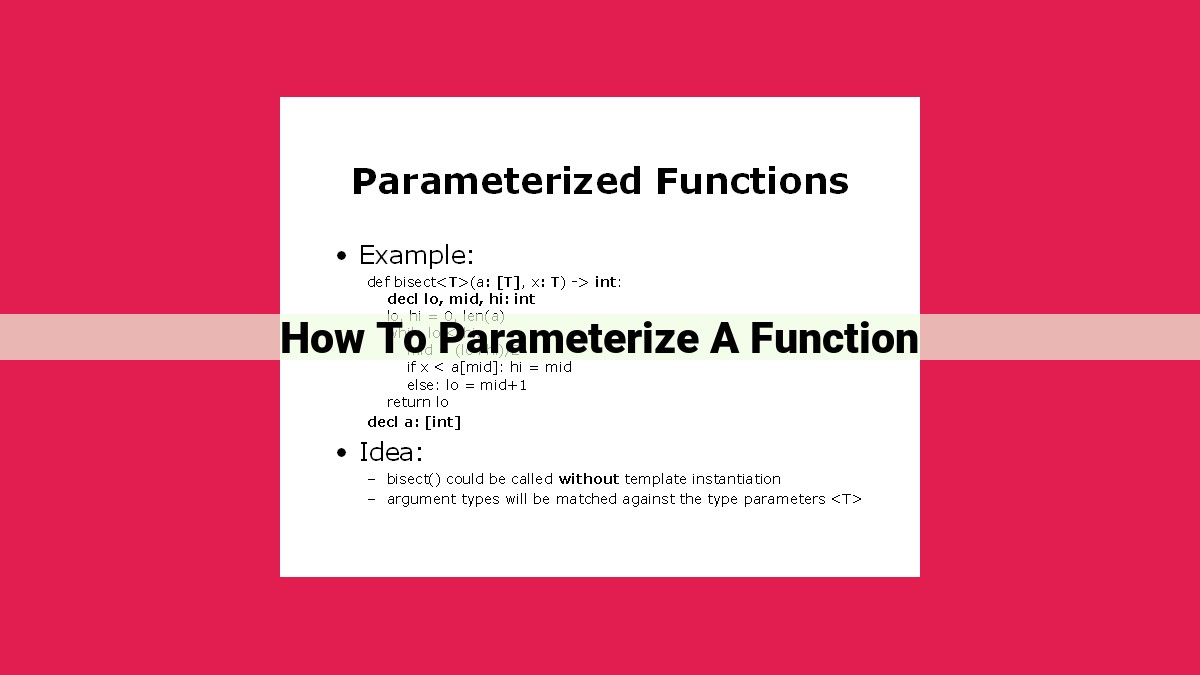
Parameterizing a function involves defining input variables (parameters) that accept values (arguments) when the function is called. These arguments can be used within the function’s code to perform specific operations or calculations. Parameters allow functions to be reused with different inputs, providing flexibility and code reusability. Default parameters, variable-length parameter lists, keyword arguments, and positional arguments are different ways to customize function inputs, enhancing their adaptability to varying scenarios.
Unlocking the Power of Functions: Understanding Function Parameters
In the world of programming, functions are the tireless workhorses that help us perform specific tasks, manipulate data, and organize our code. The key to harnessing the full potential of functions lies in understanding their parameters – the essential ingredients that allow us to personalize these tasks.
What are Function Parameters?
Parameters are special variables defined within a function’s parentheses. They act as placeholders, waiting to be filled with specific values, also known as arguments, when the function is invoked. The values of the parameters determine the behavior and output of the function, making them indispensable for customizing and controlling the function’s actions.
Syntax and Function Signatures
The function signature is the declaration of a function that specifies the names and types of its parameters. It provides a blueprint for how the function should be called, ensuring that the appropriate values are passed to the parameters. The general syntax for a function signature is:
def function_name(parameter1, parameter2, ..., parameterN):
Types of Parameters
Functions can have different types of parameters, each serving a specific purpose:
Default Parameters
Default parameters assign a predefined value to a parameter, allowing the function to be called without explicitly specifying an argument. This enhances the flexibility and convenience of function usage.
Variable-Length Parameter Lists
Variable-length parameter lists allow functions to accept an arbitrary number of arguments. These arguments are stored in a special list-like object, providing immense flexibility for handling data of varying lengths.
Keyword Arguments
Keyword arguments enable arguments to be passed by name, rather than by position. This approach offers greater clarity and readability, especially when dealing with functions with numerous parameters.
Positional Arguments
Positional arguments are passed to the function based on their position in the argument list. The order in which these arguments are passed must match the order in which they are defined in the function signature.
Customizing Function Signatures
Functions can be customized through signature customization and overloading, allowing for multiple versions of the same function with different signatures. This flexibility enhances code readability, maintainability, and extensibility.
By harnessing the power of parameters, we unlock the true potential of functions. They become versatile tools that can be tailored to our specific needs, enabling us to create efficient and elegant code that solves complex problems.
Discuss related concepts such as arguments, syntax, and function signatures.
Unlock the Power of Function Customization: A Comprehensive Guide to Function Parameters
In the world of programming, functions are essential tools that allow us to organize and reuse code. But what if we want to make our functions more versatile and adaptable? That’s where function parameters come into play.
Understanding the Basics
Function parameters are variables that hold values passed to a function when it’s called. They act like input gates, allowing us to specify what kind of data the function expects. Related concepts include:
- Arguments: The values we pass into function parameters.
- Syntax: The rules for how to declare and use function parameters.
- Function Signature: A concise representation of a function’s parameters and return type.
Exploring Function Syntax
Functions are declared with a specific syntax that includes parameters:
def function_name(parameter1, parameter2, ...):
# Function body
Parameters can be either positional (passed in a specific order) or keyword (passed by name).
Default Parameters and More
To provide default values to parameters, we can use default parameters:
def function_name(parameter1=default_value, ...):
# Function body
Variable-length parameter lists allow functions to accept an indefinite number of arguments:
def function_name(*args):
# Function body
Customizing Function Signatures
For greater flexibility, we can use function signature customization to create multiple versions of a function with different parameter combinations. Function overloading is a more advanced technique that allows us to create multiple functions with the same name but different signatures.
Benefits of Function Parameters
- Flexibility: Parameters make functions adaptable to different scenarios.
- Code Reusability: Functions with parameters can be reused for various inputs.
- Improved Readability: Parameters clarify the function’s purpose and requirements.
Function parameters are a powerful tool that unlocks the customization and versatility of functions. By understanding these concepts, you can create more robust and flexible code, making your programming journey smoother and more efficient.
Explain the syntax of functions and how parameters are used.
Function Parameters: The Key to Customizing Your Functions
In the world of programming, functions are indispensable tools that perform specific tasks. Just like a recipe requires ingredients, functions need parameters to work their magic. These parameters are like variables within a function, allowing you to tailor its behavior to your specific needs.
The syntax of a function in most programming languages looks like this:
function_name(parameter1, parameter2, ..., parameterN)
For example, let’s say you have a function called calculate_area
that calculates the area of a rectangle. It takes two parameters: length
and width
. The syntax of this function would be:
calculate_area(length, width)
When you call this function, you provide values for the parameters, like this:
result = calculate_area(5, 3)
In this case, length
is assigned the value 5 and width
is assigned the value 3. The function then uses these values to calculate the area of the rectangle, which is returned as the result.
The beauty of parameters is that they allow you to create flexible functions that can adapt to different inputs. By changing the values of the parameters, you can change the output of the function without having to rewrite the code. This makes functions highly reusable and efficient.
So, remember, next time you’re building functions, give some thought to the parameters. They are the gateway to creating powerful and versatile code that can handle a wide range of tasks.
Understanding Function Parameters: The Key to Functional Flexibility
When working with functions in programming, parameters play a crucial role in shaping how these functions behave and interact with their environment. They act as the gatekeepers to a function’s functionality, enabling it to adapt to different scenarios and tailor its behavior to specific needs.
Function Syntax and Parameters: The Ballroom of Function Calls
The syntax of functions defines the structure in which they are written. Parameters, represented by placeholders within this structure, are the variables that receive values when the function is invoked. These values, known as arguments, are passed into the function to dance with its parameters, influencing the function’s behavior.
Default Parameters: Setting the Stage for Flexibility
Default parameters, as their name suggests, provide built-in values to function parameters. When no argument is provided for a parameter, the default value steps in, ensuring that the function can still execute gracefully. This flexibility allows you to handle a wider range of scenarios without the need for additional code.
Variable-Length Parameter Lists: Embracing the Unpredictable
Variable-length parameter lists, also known as varargs, offer the ability to accept an arbitrary number of arguments. This feature proves invaluable in situations where the number of arguments is dynamic or unknown in advance. Varargs act like a catch-all, ensuring that your function can handle any surprises that come its way.
Keyword Arguments: Precision in the Dance of Function Calls
Keyword arguments allow you to pass arguments to a function by name rather than position. This precision can greatly enhance code readability and reduce the chances of errors. Instead of relying on the order of arguments, you can explicitly specify which parameter receives each value, ensuring that your intentions are crystal clear.
Positional Arguments: The Traditional Dance Partners
Positional arguments, the more traditional approach, rely on the order in which arguments are passed to a function. Each parameter must receive a value in the correct position, or else the function may falter. While this may seem restrictive, positional arguments offer predictability and can often simplify code.
Function Overloading and Signature Customization: Adapting to Every Beat
Function overloading allows you to create multiple versions of a function with the same name but different parameter signatures. This flexibility enables you to tailor the function to specific scenarios, providing specialized behavior for each need. Similarly, signature customization gives you the power to change the parameter order, types, and even default values, further enhancing your ability to control and adapt function behavior.
In the ever-evolving world of programming, parameters stand as the silent heroes, empowering functions with flexibility, adaptability, and precision. By understanding the nuances of function parameters, you can elevate your code, transforming it from a mere tool into a responsive and versatile performer on the stage of your application.
Define default parameters and show how they can be used to provide default values to function arguments.
Default Parameters: Simplifying Function Calls
In the world of programming, functions are like tools that perform specific tasks. Imagine having a wrench that only fits one type of bolt. While convenient for that particular bolt, it becomes limiting when you need to work with other sizes. Similarly, functions with hard-coded parameters can be inflexible. That’s where default parameters come in, providing a way to make functions more versatile.
Default parameters are like запасные планы for functions. They allow you to specify a default value for a parameter, which is used if the caller doesn’t provide a specific value. This eliminates the need for conditional checks within the function, simplifying the code and making it more readable.
For example, consider a function that calculates the area of a rectangle. Typically, you would need to provide both the length and width as parameters. However, if you know that the length is usually fixed, you can set a default value for it:
def rectangle_area(width, length=10):
return width * length
Now, when you call this function without specifying the length, it will use the default value of 10. This provides greater flexibility and makes your code more concise and maintainable.
Default parameters can also enhance the readability of your code. By clearly specifying the default values, you convey the intended usage of the function without the need for additional comments or documentation. This improves the overall understanding and reusability of your codebase.
In summary, default parameters are a powerful tool for making functions more flexible and user-friendly. They allow you to specify backup values for parameters, eliminating the need for conditional checks and enhancing the readability of your code. Embrace default parameters to simplify your programming tasks and create more adaptable and maintainable code.
Explain the syntax and function signature of default parameters.
How to Parameterize a Function: A Comprehensive Guide
Understanding Function Parameters
In programming, functions are like recipes that take inputs (known as arguments) and produce outputs. Parameters are placeholders in these recipes, representing the variables that will receive the input values. They help you create versatile functions that can handle different sets of data.
Function Syntax and Parameters
The syntax of a function includes its name, parameter list, and return type. Parameters are enclosed in parentheses, separated by commas. For example:
def greet(name):
return f"Hello, {name}!"
In this function, name
is the parameter that will receive the name of the person to be greeted.
Default Parameters
Default parameters allow you to provide a default value to a function argument. This is useful when you want a function to behave a certain way if no argument is passed.
The syntax for a default parameter is:
def greet(name="Stranger"):
return f"Hello, {name}!"
In this example, if no name is passed when the function is called, it will use the default value “Stranger”.
Variable-Length Parameter Lists
Variable-length parameter lists allow you to accept an indefinite number of arguments. This is handy when you’re working with data sets of unknown size.
The syntax for a variable-length parameter list is:
def average(*numbers):
return sum(numbers) / len(numbers)
In this function, *numbers
represents a variable-length list that can contain any number of numeric arguments. It’s useful for calculating averages or other statistics.
Variable-Length Parameter Lists: Embracing Flexibility in Function Arguments
Imagine you’re hosting a party and you want to invite people to bring dishes to share. Instead of specifying a fixed number of dishes, you decide to allow guests to bring as many dishes as they like. This flexibility ensures that you’ll have an abundance of food without being limited by a rigid number.
Similarly, in programming, variable-length parameter lists offer the same flexibility in function arguments. Let’s explore how these lists empower you to handle an undetermined number of arguments in your functions.
The Syntax of Variable-Length Parameter Lists
To create a function with a variable-length parameter list, simply use three dots (…) followed by the parameter name. For example:
def sum_numbers(...numbers):
total = 0
for num in numbers:
total += num
return total
In this function, ...numbers
is the variable-length parameter list. It allows you to pass an arbitrary number of arguments when you call the function.
Calling Functions with Variable-Length Parameter Lists
When calling a function with a variable-length parameter list, you can pass as many arguments as you need. The function will automatically create a tuple containing these arguments and assign it to the variable-length parameter.
result = sum_numbers(1, 2, 3, 4, 5)
In this example, the sum_numbers
function is called with five arguments. The function will create a tuple (1, 2, 3, 4, 5)
and assign it to the ...numbers
parameter.
Advantages of Variable-Length Parameter Lists
- Flexibility: You can handle functions with an unpredictable number of arguments.
- Code Reusability: You can write functions that can be used for various scenarios with varying numbers of arguments.
- Simplicity: Variable-length parameter lists provide a convenient way to handle _不定 number of arguments without complex code constructs.
Remember: When working with variable-length parameter lists, it’s important to consider the following:
- Define the default value for the parameter to handle cases when no arguments are passed.
- Use this feature judiciously to avoid creating functions with too many or inconsistent arguments.
Variable-Length Parameter Lists: A Flexible Tool for Argument Handling
Imagine you’re planning a party and need to create a guest list. You know there will be a varying number of attendees, so you can’t fix a specific number of guests in your list. That’s where variable-length parameter lists come in—they allow you to handle an indefinite number of arguments, just like your expanding guest list.
In programming, variable-length parameter lists are denoted using asterisks (). For example, if you have a function called add_numbers
that takes multiple numbers and calculates their sum, you can use a variable-length parameter list to accept any number of arguments. The syntax looks like this:
def add_numbers(*args):
# ...
Here, the *args
represents a tuple that collects all the arguments passed to the function. You can then iterate over the args
tuple to perform operations on each individual argument. This flexibility gives you the freedom to handle varying amounts of data without hard-coding the number of parameters.
Function Signature with Variable-Length Parameter Lists
The function signature of a function with variable-length parameter lists also includes the asterisk (*). For the add_numbers
function, the signature would be:
def add_numbers(*numbers) -> int:
# ...
The *numbers
part indicates that the function accepts a variable number of arguments, and the -> int
part specifies that the function returns an integer. This clear representation helps other developers understand how your function works.
By using variable-length parameter lists, you gain flexibility in function design and can easily handle data of varying sizes. It’s a powerful tool that allows you to write adaptable and reusable code, making your programming life much easier.
Keyword Arguments: Passing Arguments by Name
In the vast realm of programming, functions reign supreme as powerful tools for organizing and executing reusable code. To empower these functions, we often pass them arguments, injecting them with the data they need to perform their magic. While positional arguments, where the order of arguments matters, have their place, behold the allure of keyword arguments – a paradigm shift that grants you the freedom to pass arguments by name.
Keyword arguments shine when you want to enhance the readability, maintainability, and flexibility of your code. By explicitly specifying the name of the argument you’re passing, you’re removing the ambiguity inherent in positional arguments. This naming convention makes it crystal clear what data is being passed to which parameter, regardless of its position in the function call.
Consider this example:
def greet(name, age):
print("Hello, my name is {}, and I'm {} years old.".format(name, age))
To invoke this function using positional arguments, you would need to supply the values in the correct order:
greet("John", 30)
However, with keyword arguments, you gain the flexibility to specify the argument names explicitly:
greet(age=30, name="John")
In this case, the order of the arguments doesn’t matter – the function will still correctly assign the values to their intended parameters. This naming convention becomes even more crucial when dealing with functions that accept a large number of arguments.
By embracing keyword arguments, you not only enhance the clarity of your code but also open the door to powerful features such as function overloading and signature customization. These advanced techniques allow you to define multiple versions of a function with different sets of parameters, further enhancing the versatility and reusability of your code.
How to Parameterize a Function: A Comprehensive Guide
Understanding the Essence of Function Parameters
In the realm of programming, functions are invaluable tools for organizing and structuring code. Parameters are the essential ingredients that functions require to execute specific tasks and produce desired outputs. They serve as placeholders for data that is passed into the function when it is called.
Function Syntax and the Role of Parameters
The syntax of a function defines its structure and the way in which parameters are used. Typically, a function declaration includes the function name, followed by parentheses containing a list of parameters. These parameters are separated by commas and can have specific data types associated with them.
For instance, consider the following function:
def calculate_area(width, height):
return width * height
In this function, width
and height
are the parameters. When this function is called, it expects two arguments to be passed into these parameters, representing the width and height of a rectangle.
Default Parameters and Their Convenience
Default parameters provide a convenient way to assign default values to function arguments. This means that if no value is provided for a parameter when the function is called, the default value will be used instead.
For example:
def greet(name="John Doe"):
print(f"Hello, {name}!")
In this function, the name
parameter has a default value of "John Doe"
. If the function is called without specifying a name, it will use this default value and print "Hello, John Doe!"
.
Variable-Length Parameter Lists: Accepting the Unknown
Variable-length parameter lists allow functions to accept an arbitrary number of arguments. These arguments are stored in a tuple or list within the function.
Consider the following function:
def print_all(*args):
for arg in args:
print(arg)
In this function, the *args
parameter accepts an arbitrary number of arguments. When the function is called, these arguments are stored in the args
tuple, which can then be iterated over.
Keyword Arguments: Passing by Name
Keyword arguments provide a way to pass arguments to a function by name instead of by position. This makes it easier to identify the purpose of each argument, especially when dealing with complex functions.
For instance:
def format_name(first_name, last_name):
return f"{first_name} {last_name}"
If we call this function using positional arguments, the order in which the arguments are passed is crucial:
formatted_name = format_name("Jane", "Doe") # Correct
However, using keyword arguments, we can specify the arguments by name, regardless of their order:
formatted_name = format_name(last_name="Doe", first_name="Jane") # Also correct
Positional Arguments: Maintaining the Order
Positional arguments are passed to a function based on their position in the function call. The first argument is assigned to the first parameter, the second argument to the second parameter, and so on.
It is important to maintain the correct order of positional arguments when calling a function, as changing the order can lead to unexpected behavior.
Consider the following function:
def sum_numbers(a, b, c):
return a + b + c
If we call this function with the arguments in the wrong order, we will get an incorrect result:
result = sum_numbers(c=1, b=2, a=3) # Incorrect
Function Overloading and Signature Customization
Function overloading allows us to create multiple versions of a function with the same name but different argument lists (signatures). This is useful when we want to provide different functionalities for the same logical operation.
For instance, we could have two versions of the sum_numbers
function: one that takes three arguments and one that takes two arguments.
def sum_numbers(a, b, c=0):
return a + b + c
def sum_numbers(a, b):
return a + b
Function signature customization allows us to change the signature of a function dynamically based on the arguments passed to it. This can be achieved using type annotations and the *
and **
operators.
For example:
def calculate(a: int, b: int) -> int:
return a + b
def calculate(a: float, b: float) -> float:
return a + b
In this example, the calculate
function can accept two integers or two floats as arguments, depending on the types of the arguments passed to it.
Positional Arguments: Passing Arguments by Position
In the world of programming, functions are like workers who perform specific tasks. They accept inputs, process them, and return results. Parameters are like the instructions handed to these workers, telling them what specific tasks to perform.
Positional arguments are a type of function parameter that you pass based on their position in the function call. Think of it like a lineup of arguments, where each one has its own designated spot. When you call a function with positional arguments, you’re specifying the values for these arguments in the order they appear in the parameter list.
For example, let’s say we have a function called calculate_area()
that takes two arguments: length
and width
. To call this function using positional arguments, you would provide the length first, followed by the width.
calculate_area(5, 3) # Passing 5 as length and 3 as width
In this call, the positional argument 5
is assigned to the length
parameter, and the positional argument 3
is assigned to the width
parameter.
Positional arguments are straightforward and easy to use, especially when the order of the arguments is clear. However, if the order is not obvious or if you want to pass arguments out of order, you can use named arguments instead.
How to Parameterize a Function: A Comprehensive Guide
Understanding Function Parameters
Parameters are crucial elements of functions that act as placeholders for values or arguments passed into the function. They determine the input that the function needs to execute and provide the flexibility to use the function in various scenarios.
Function Syntax and Parameters
Functions are defined using a specific syntax that includes parameters enclosed within parentheses. The function’s signature, which consists of the parameter list and return type, plays a vital role in identifying and calling the function. In Python, for example, a function with a single parameter x
would be declared as def function(x)
.
Default Parameters
Default parameters allow you to assign default values to parameters, making them optional. This is useful when certain parameters have frequently used values that you want to set by default, eliminating the need to pass them explicitly. In the example above, you could define the default value of x
as 1
using the following syntax: def function(x=1)
.
Variable-Length Parameter Lists
Variable-length parameter lists, represented by *args
in Python, enable you to pass an indeterminate number of arguments to a function. This flexibility is valuable when you don’t know how many arguments to expect, for example, when aggregating values from a user’s input.
Keyword Arguments
Keyword arguments provide a way to pass arguments by name rather than by position. This approach offers more clarity and readability, especially when working with a large number of parameters. In Python, keyword arguments are preceded by the argument name, such as def function(name="John")
.
Positional Arguments
Positional arguments are the traditional way of passing arguments to functions. They are based on the positional order of the parameters in the function signature. For example, the function def add(a, b)
would expect two positional arguments, a
and b
, to be passed in that order during the function call.
Function Overloading and Signature Customization
Function overloading and signature customization allow you to create multiple versions of a function with different signatures, enabling you to handle different combinations of inputs. Function overloading involves defining multiple functions with the same name but distinct signatures, while signature customization allows you to modify the parameter list of a single function. These techniques provide flexibility and adaptability when designing complex functionalities.
How to Parameterize a Function: A Comprehensive Guide
Understanding Function Parameters
In the realm of programming, functions are essential tools for encapsulating code and making it reusable. One crucial aspect of defining functions is using parameters, which allow you to customize your functions to handle different scenarios. Parameters are variables that can be passed into a function when it’s called, providing input to the function’s execution.
Function Syntax and Parameters
The syntax of a function definition includes the def
keyword, the function name, the parameter list in parentheses, and the function body. Parameters are declared within the parentheses, separated by commas. The function body is where the code that processes the input and performs the desired actions is written.
Functions can have multiple parameters, with each parameter having a unique name and type. When a function is called, arguments are provided to match each parameter. The arguments are passed in the same order as the parameters are declared.
Default Parameters
Default parameters provide a convenient way to assign default values to function arguments. When an argument is not provided during a function call, the default value is used instead. Default parameters are defined by assigning a value to the parameter name within the function definition.
For example:
def greet(name, message="Hello"):
print(f"{message} {name}!")
If the greet
function is called with only one argument, the default value for message
will be used. This allows you to make your functions more flexible and adaptable to different use cases.
Variable-Length Parameter Lists
Variable-length parameter lists allow you to create functions that can accept an arbitrary number of arguments. This is achieved using the asterisk *
before the parameter name. The arguments passed to the function are stored in a tuple, enabling you to process multiple inputs in a single function call.
For example:
def sum_numbers(*numbers):
total = 0
for number in numbers:
total += number
return total
The sum_numbers
function can be called with any number of arguments, and it will calculate the sum of all the provided numbers.
Keyword Arguments
Keyword arguments allow you to pass arguments to a function by name rather than by position. This provides greater flexibility and readability, especially when working with functions with multiple parameters. Keyword arguments are specified by preceding the argument name with a double colon **
.
For example:
def greet(name, message):
print(f"{message} {name}!")
The above function can be called using keyword arguments as follows:
greet(message="Good morning", name="John")
Positional Arguments
Positional arguments are the most common type of arguments and are passed to a function in the same order as the parameters are declared. Positional arguments do not have names associated with them, and the order in which they are passed is crucial.
For example:
def greet(name, message):
print(f"{message} {name}!")
The following function call provides positional arguments:
greet("John", "Good morning")
Function parameters are a fundamental concept in programming and allow you to create flexible and reusable code. By understanding the different types of parameters, such as default parameters, variable-length parameter lists, keyword arguments, and positional arguments, you can harness their power to enhance the functionality and readability of your code.
Understanding Function Overloading for Parameterization Bliss
The Puzzling World of Functions and Parameters
In the realm of coding, functions are like secret agents, performing specific tasks under the hood. To effectively carry out these missions, they rely on parameters, which are like the instructions they follow. Parameters tell the function what data to use and how to process it.
Syntax Symphony: A Peek into Function Structure
When we define a function, we specify its parameters within the parentheses after its name. These parameters, like placeholders, define the data that the function expects to receive. The syntax looks something like this:
function_name(parameter1, parameter2, ...)
Default Parameters: When Flexibility Trumps Rigidity
Sometimes, we want our functions to be adaptable, capable of handling missing data. Here’s where default parameters step in. They provide a backup value for parameters that aren’t explicitly specified in the function call.
Variable-Length Parameter Lists: Embracing the Unknown
In the world of coding, flexibility is key. Variable-length parameter lists allow functions to accept any number of arguments, providing us with the freedom to handle data that varies in quantity.
Keyword Arguments: Unlocking the Power of Named Data
Keyword arguments introduce an elegant way to pass data to functions. Instead of relying on positional order, we can explicitly specify the names of parameters when making function calls. This enhances code readability and reduces the risk of errors.
Positional Arguments: The Classic Approach
Positional arguments, on the other hand, rely on the order of parameters in the function call. We pass data in the same order as the parameters are defined within the function. This approach is straightforward but can be prone to errors if the order is incorrect.
Function Overloading: A Symphony of Signatures
Imagine a situation where we need multiple versions of the same function, each with a different set of parameters. This is where function overloading comes into play. It allows us to define multiple functions with the same name but different signatures (i.e., different parameter lists).
Understanding function parameters, including default parameters, variable-length parameter lists, keyword arguments, positional arguments, function signature customization, and function overloading, empowers us to create flexible and efficient code. By embracing these concepts, we can unleash the full potential of functions, ensuring they fulfill their missions with precision and adaptability.