Ultimate Guide To Iterating Backwards In Python: Techniques And Best Practices
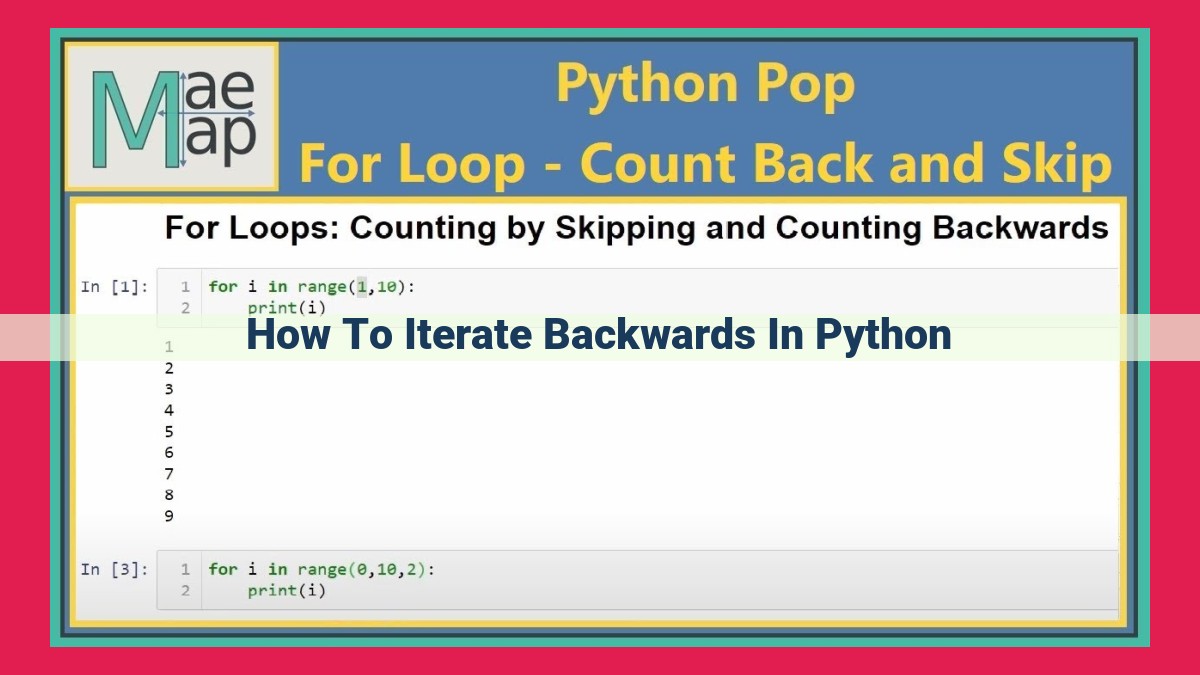
Iterating backwards in Python allows you to traverse a sequence in reverse order. This is useful for tasks like reversing a list or string, processing data from the last element, or analyzing data in a reverse chronological order. Common methods include slicing with negative strides, using the reversed() function for lazy iteration, creating reversed lists using list comprehensions, and employing the itertools.islice() function for partial reversed iteration. The best approach depends on factors like performance, memory consumption, and the desired level of control over the iteration process.
Mastering Reversed Iteration: Techniques and Use Cases
In the realm of programming, traversing through data structures in reverse order holds significant importance. Reversed iteration empowers us to access elements from the end of a sequence, often leading to efficient solutions and intuitive code.
Why Reversed Iteration?
Reversed iteration finds applications in a wide array of scenarios. Consider a list of recent transactions in a banking application. To display them chronologically, starting from the latest, we need to iterate over the list in reverse order. Similarly, in data analysis, processing datasets from the end can provide valuable insights.
Methods for Reversed Iteration
Various techniques exist to facilitate reversed iteration in Python. Let’s explore them one by one:
Slicing with Negative Strides
The simplest way to reverse a sequence is through slicing with negative strides. For instance, to reverse a list my_list
, we can use my_list[::-1]
. The negative stride (-1) indicates that we should iterate over the list from the end.
Reversed() Function
Python offers a built-in reversed()
function that returns an iterator over a sequence in reversed order. Unlike slicing, reversed()
does not create a new copy of the sequence, making it memory-efficient.
List Comprehension
List comprehensions provide a concise way to reverse iterate a sequence. The syntax is similar to a regular list comprehension, but with the elements reversed: [element for element in my_list reversed]
.
Itertools.islice() Function
The itertools.islice()
function allows for partial reversed iteration. It takes three arguments: the sequence to be iterated over, the start index (negative to start from the end), and the stop index (negative to indicate the number of elements from the end).
Choosing the Right Technique
The choice of reversed iteration technique depends on the specific requirements. For simple and immediate reversal of a sequence, slicing is sufficient. If memory efficiency is crucial, the reversed()
function is recommended. List comprehensions offer a concise solution, while itertools.islice()
enables partial reversed iteration.
Understanding the nuances of reversed iteration is essential for efficient and elegant programming. The techniques discussed in this blog post empower programmers to navigate sequences from the end, unlocking new possibilities and simplifying complex operations.
Reverse Iteration in Python: A Step-by-Step Guide
In the world of Python, you may often encounter scenarios where you need to iterate over a sequence in reverse order. Reversed iteration offers a powerful way to achieve this, opening up a realm of possibilities for data analysis, text processing, and more. This blog post will embark on a journey through various techniques to perform reverse iteration in Python, empowering you with the knowledge to navigate your data with ease.
Let’s delve into the concept of slicing, a versatile method for manipulating sequences in Python. Slicing allows you to extract specific portions of a sequence using a syntax that combines the start, end, and stride values. Negative strides play a crucial role in reverse iteration, as they enable you to traverse a sequence backward.
For instance, consider the following list:
my_list = [1, 2, 3, 4, 5]
To iterate over this list in reverse order using slicing, you can use the following syntax:
for i in my_list[::-1]:
print(i)
The expression [::-1]
is a slice that starts at the end of the list (index -1), ends at the beginning (index 0), and takes steps of -1, effectively reversing the order of elements. As a result, the output of the code above will be:
5
4
3
2
1
Slicing provides a straightforward and efficient way to reverse iterate over a sequence. It is particularly useful when you need to create a new reversed sequence or perform in-place reversal of an existing one.
Reversed() Function:
- Introduce the reversed() function and its benefits.
- Demonstrate how to use reversed() to iterate over a sequence in reverse order lazily.
Harnessing the Power of Python: Mastering Reversed Iteration
In the realm of Python programming, traversing sequences in reversed order is a common task. Be it arrays, lists, or strings, the ability to iterate through elements from end to start provides flexibility and efficiency in various scenarios.
The Arcane Art of Reversed Iteration
Reversed iteration is a technique that allows you to traverse a sequence backwards, element by element. This invaluable tool has found widespread use in domains ranging from data analysis to text processing.
Slicing: A Simple Yet Effective Approach
Python’s slicing mechanism offers a straightforward way to reverse iterate a sequence. By specifying negative strides, you can access elements from the end towards the beginning. For instance:
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1] # Slice with a stride of -1
In this example, reversed_list
will contain the elements [5, 4, 3, 2, 1]
.
Unveiling the Reversed() Function: A Lazy Iterator
Python’s reversed()
function provides a lazy iterator that allows you to iterate over a sequence in reverse order. Unlike slicing, which creates a new list, reversed()
retains the original sequence and generates elements on demand. This memory-efficient approach is particularly useful for large sequences.
for item in reversed(my_list):
print(item)
The code above will print 5 4 3 2 1
without modifying my_list
.
Exploring Python’s Reversed Iteration Techniques: A Guide for Navigating Sequences Backwards
In the realm of Python programming, it often becomes necessary to traverse sequences in reverse order. This reversed iteration can help you process data from the last element of a list or string to the first, making it a valuable technique in various scenarios. Let’s embark on a journey to explore some of the most commonly used methods for reversed iteration in Python.
Slicing: A Direct Approach
One straightforward way to reverse iterate a sequence is through slicing. By specifying a negative stride, you can effectively flip the order of elements. For instance, to reverse a list named “numbers,” you can use:
reversed_numbers = numbers[::-1]
This results in a new list “reversed_numbers” that contains the elements of “numbers” in reverse order.
Reversed() Function: A Lazy Solution
A more flexible option is the reversed() function. It returns a reverse iterator, which generates elements lazily as you iterate over it. This saves memory, especially when dealing with large sequences. To use reversed(), simply apply it to your sequence:
for number in reversed(numbers):
# Process number in reverse order
List Comprehension: A Concise Alternative
List comprehensions offer a concise way to perform reversed iteration. By combining comprehensions with negative indexing, you can create a new list with the elements in reverse order:
reversed_numbers = [number for number in numbers[::-1]]
Itertools.islice() Function: Partial Reversed Iteration
The itertools.islice() function allows you to perform partial reversed iteration. This is useful when you need to access only a portion of the sequence in reverse order. To use islice(), specify the starting index (negative for reversed iteration) and the number of elements you want to retrieve:
reversed_slice = list(itertools.islice(reversed(numbers), 0, 3))
In this example, “reversed_slice” will contain the first three elements of “numbers” in reverse order.
Choosing the appropriate reversed iteration technique depends on your specific requirements. If you need a direct reversal of a small sequence, slicing is efficient. For larger sequences, reversed() provides lazy iteration, saving memory. List comprehensions offer a concise approach, while itertools.islice() allows for partial reversed iteration. Understanding these techniques will enhance your ability to navigate Python sequences in reverse order effectively.
Reverse Iteration in Python: A Comprehensive Guide
Reversed iteration is a crucial technique in Python that allows you to traverse a sequence in reverse order. It finds widespread use in real-world applications, such as data analysis, string manipulation, and more. This guide will explore various methods for reversed iteration in Python, providing a comprehensive understanding of their functionality and use cases.
Slicing
Slicing is a versatile tool in Python that enables you to extract a subset of a sequence. By specifying negative strides in slicing, you can achieve reversed iteration. For instance, my_list[::-1]
produces a reversed copy of my_list
. Similarly, my_string[::-1]
reverses a string.
Reversed() Function
The reversed()
function is a powerful built-in that returns an iterator that yields elements of a sequence in reverse order. Unlike slicing, reversed()
iterates lazily, saving memory and computational resources. To iterate over a sequence in reverse using reversed()
, simply wrap it around the sequence.
List Comprehension
List comprehensions offer a concise alternative to reversed()
. They allow you to create a new list by applying an expression to each element of an existing sequence. To reverse iterate using list comprehensions, simply reverse the range of the loop.
Itertools.islice() Function
Partial Reversed Iteration
The itertools.islice()
function provides an additional option for partial reversed iteration. It extracts a specified number of elements from the reversed end of a sequence. Using negative values for the start and stop parameters, you can define a range for partial reversed iteration.
For example, islice(my_list, -3, None)
returns the last three elements of my_list
in reversed order. Similarly, islice(my_string, -5, None)
reverses the last five characters of my_string
.
This guide has covered a range of techniques for reversed iteration in Python. Each method offers unique advantages, making it essential to choose the approach that best suits your specific requirements. Whether you need a full or partial reversed iteration, lazy evaluation or a concise syntax, these methods empower you to manipulate sequences with ease and efficiency. Now, you can tackle your next Python project with confidence, knowing you have the tools and understanding to iterate in reverse with precision.