How To Read A Text File In C++: A Comprehensive Guide For Beginners
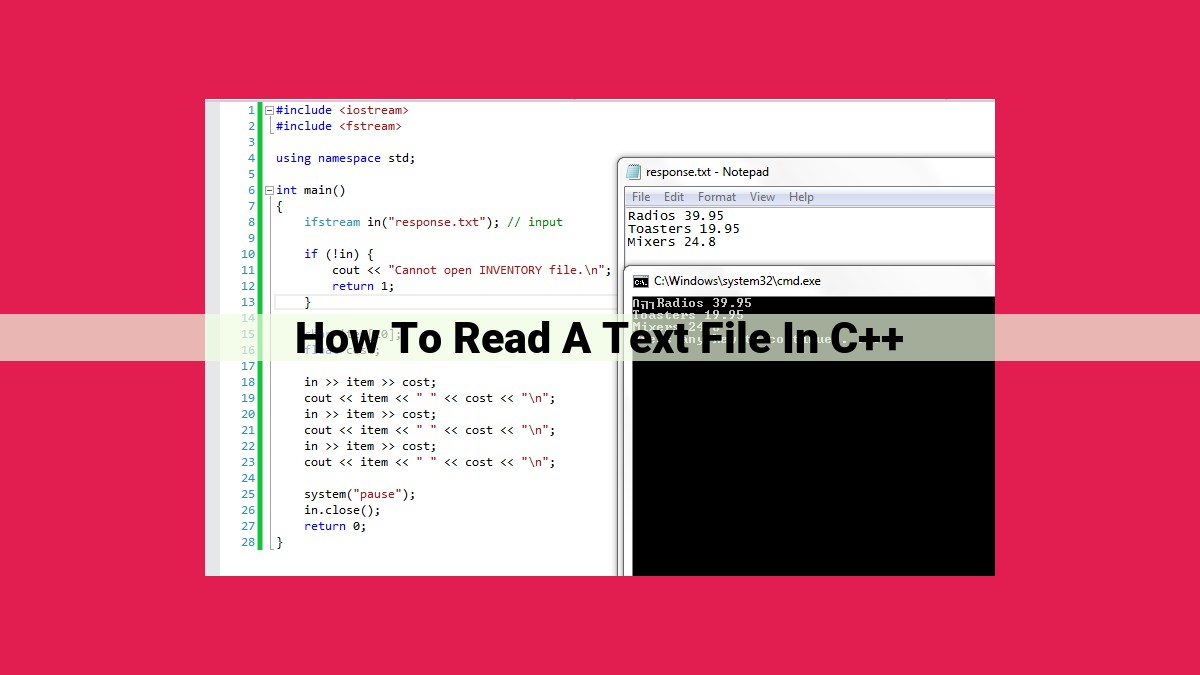
To read a text file in C++, use the ifstream
class to establish a connection to the file. Ensure successful file opening with is_open()
, then read individual characters with get()
or entire lines with getline()
. Stream extraction (>>
) can be employed to extract data into variables. Iterate through the file contents using a while
loop, processing each line.
Understanding File Handling in C++
In the realm of programming, file handling empowers us to interact with files, store data, and perform various operations on them. In C++, we can leverage file streams to establish this communication between the program and files. These streams act as conduits, allowing us to read and write to files.
Input streams enable us to access data from files, treating them as a source of information. Output streams, on the other hand, facilitate data writing to files, serving as a destination for our program’s output. These streams simplify file handling, providing a structured way to interact with files.
Using ifstream to Read from a Text File
Embark on a journey through the realm of file handling in C++, where you’ll master the art of reading text files. Our trusty companion on this adventure is the ifstream
class, a gateway to unlocking the secrets within your text files.
Introducing the ifstream
Class
Picture ifstream
as a dedicated reader, eagerly awaiting the chance to delve into your text files. It’s an input stream that reads characters and strings from files, allowing you to extract the precious data stored within.
Opening the Door to Your Text File
Before you can read from a file, you must first establish a connection using the open()
function. Think of it as knocking on the file’s door, requesting permission to enter. When choosing the file path, ensure it’s accurate and leads to the desired file.
ifstream fileObject;
fileObject.open("my_text_file.txt");
Verifying Your Connection
Once you’ve knocked, it’s crucial to check if the file has opened successfully. This is where is_open()
comes in handy. It acts as your checkpoint, confirming whether the connection was established.
if (fileObject.is_open()) {
// File opened successfully. Proceed with reading.
} else {
// File opening failed. Handle the error.
}
Reading Individuals: Using get()
Imagine the get()
function as a careful inspector, meticulously examining each character in your file. It retrieves these characters one by one, allowing you to process them character by character or store them in an array.
Reading Lines: Unveiling getline()
For a broader view, getline()
steps in. It acts as an efficient reader, capturing entire lines of text from your file. As it navigates through the file, it reads one line at a time, opening up endless possibilities for line-by-line processing.
Opening a Text File with open()
When embarking on our quest to read a text file in C++, the first step is to establish a connection to the file. This is achieved through the open()
function, a powerful tool in our file handling arsenal.
Before invoking open()
, we must specify the path to our desired text file, a string that pinpoints its location. Additionally, we need to define the file mode, which dictates how we intend to interact with the file.
File modes are crucial for controlling file access. The most common modes are:
ios::in
: Opens the file for reading.ios::out
: Opens the file for writing, overwriting any existing content.ios::app
: Opens the file for writing, appending new content to the end.
Specifying the file mode ensures proper file handling. For instance, attempting to read from a file opened in ios::out
mode will result in unexpected behavior.
To open a text file, simply invoke open()
with the file path and desired mode:
ifstream input_file;
input_file.open("myfile.txt", ios::in);
This code opens the file "myfile.txt"
for reading. However, before proceeding, it’s prudent to verify that the file was successfully opened.
Verifying File Status with is_open()
In the realm of file handling in C++, ensuring that a file has been successfully opened is crucial. This is where the humble yet indispensable is_open()
function comes to the rescue. Upon opening a file, it’s imperative to verify its status to prevent unexpected errors and guarantee seamless data transfer.
The is_open()
function, like a watchful guardian, stands ready to provide valuable insight into the file’s availability for reading. It returns a boolean value: true
if the file has been successfully opened and is ready for action; false
if the opening attempt has failed due to various reasons, such as incorrect file paths, insufficient permissions, or system limitations.
By harnessing the power of is_open()
, programmers can avoid potential pitfalls and gracefully handle file-related operations. It acts as a safety net, preventing coding headaches and ensuring that the file handling process proceeds without any hiccups.
Reading Characters Using get()
Continuing our exploration into the realm of file handling in C++, we now delve into the depths of character-level file reading using the get()
function. This function allows us to read individual characters from a text file, opening up a myriad of possibilities for data extraction and processing.
The get()
function works by reading a single character from the input file stream and storing it in a character variable. This process enables us to sequentially retrieve each character from the file, allowing us to inspect and manipulate its contents at a granular level.
To effectively utilize the get()
function, we must first open the file in read mode using the open()
function. Once the file is successfully opened, we can use the get()
function to read the first character. If there are more characters in the file, we can continue reading them one by one until we reach the end-of-file indicator.
The get()
function is particularly useful when we need to process individual characters or when we want to parse the file’s contents into a specific format. For instance, we could use the get()
function to read a text file containing comma-separated values and extract each field into a structured data format.
Overall, the get()
function provides a powerful mechanism for reading characters from a text file in C++. By understanding its functionality and application, we can harness the full potential of file handling and unlock a wide range of data processing capabilities.
Reading Lines with getline()
Embark on a Literary Adventure with getline()
In our quest to uncover the secrets of file handling in C++, we encounter a powerful tool: the getline()
function. This trusty companion allows us to effortlessly read entire lines of text from a file, paving the way for limitless possibilities.
String Theory and File Reading
getline()
is intimately intertwined with the world of string I/O. Strings, the building blocks of text, become our allies as we delve into the depths of file reading. By harnessing getline()
, we can effortlessly extract lines of text and store them as strings, ready for further exploration and analysis.
A Glimpse into the getline()
Mechanism
Think of getline()
as a literary explorer, venturing into the vast expanse of a text file. With each line it traverses, it captures the entire sequence of characters, including spaces, punctuation, and even line breaks. The result? A pristine string, mirroring the original line in the file, eagerly awaiting our attentive analysis.
Mastering the getline()
Syntax
To wield the power of getline()
, simply invoke it with a reference to your input stream and a string variable to store the extracted line:
std::string line;
std::getline(input_stream, line);
And like magic, the line of text is plucked from the file and gracefully nestled within the line
variable, ready to be scrutinized or processed as you desire.
The Elegance of getline()
in Action
Imagine a text file brimming with lines of poetic verse. Using getline()
, we can traverse each line, capturing its ethereal essence in a collection of strings. These strings, like puzzle pieces, can be assembled to recreate the original masterpiece or dissected to reveal hidden patterns and insights.
With getline()
as our literary guide, we unlock the ability to traverse text files with ease. Its elegance and simplicity empower us to explore the written word in C++, extracting lines of wisdom, knowledge, and artistic beauty. Embrace the power of getline()
and let your literary adventures soar to new heights.
Reading Text Files in C++: Using >> for Stream Extraction
In our quest to master the art of file handling, we’ve delved into the world of input/output streams. The ifstream
class has been our trusty companion for reading text files, and now, we’ll unveil another powerful tool: the >>
operator.
This enigmatic symbol may seem unassuming, but it possesses the ability to extract data from files and transform it into usable information. The magic lies in its ability to convert data types. Let’s explore how this works:
Imagine you’re reading a text file that contains numbers. Each number is stored as a string of characters, but you want to store it as an integer in your program. The >>
operator steps in here, working behind the scenes to convert the string to an integer. It’s like having a secret decoder ring that translates file contents into the language your program understands.
But the >>
operator’s powers extend beyond numbers. It can also handle characters, strings, and even user-defined types. It’s an incredibly versatile tool that simplifies the process of reading files and extracting meaningful data.
So, how do you use this magical operator? It’s as simple as assigning the extracted data to a variable. For example, to read a line of text and store it in a string, you would write:
string line;
ifstream file("my_file.txt"); // Open the file for reading
file >> line; // Read a line of text into the 'line' variable
In this code, the >>
operator extracts the next line of text from the file and assigns it to the line
variable. It’s like a conveyor belt that transports data from the file into your program.
Stream extraction, enabled by the >>
operator, is an essential tool for reading text files in C++. It allows you to convert data types on the fly, making it easy to extract and process information from files. So, embrace the power of >>
and unlock the secrets hidden within your text files.
Looping Through File Contents with while
Once we have successfully opened a text file and verified its status, we can proceed to read its contents. One way to do this is by using a while
loop.
A while
loop is a control structure that allows us to execute a block of code repeatedly until a certain condition is met. In this case, we can use a while
loop to read each line of text from the file.
The following code snippet demonstrates how to use a while
loop to read the contents of a text file line by line:
while (!file.eof()) {
string line;
getline(file, line);
// Process the line here...
}
In this code, the while
loop continues as long as the eof()
method of the file stream object file
returns false
. The eof()
method returns true
when the end of the file has been reached.
Inside the loop, the getline()
function is used to read a line of text from the file and store it in the line
string variable. The getline()
function reads characters from the file until it encounters a newline character (\n
).
Once a line of text has been read, we can process it as needed. This could involve parsing the line, extracting specific information, or performing some other operation.
The while
loop continues to read lines of text from the file until the end of the file is reached. At that point, the eof()
method will return true
and the loop will terminate.